Power-Up your JavaScript
with Flow
The language we so much
[] + [] // ''
[] + {} // [object Object]
{} + [] // 0
{} + {} // NaN
true + true === 2 // true
true - true === 0 // true
true === 1 // false
Static vs. Dynamic
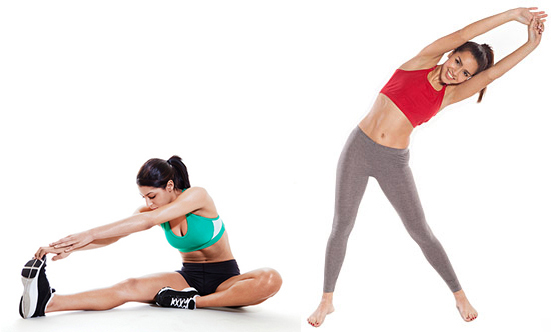
Gradual typing in JavaScript
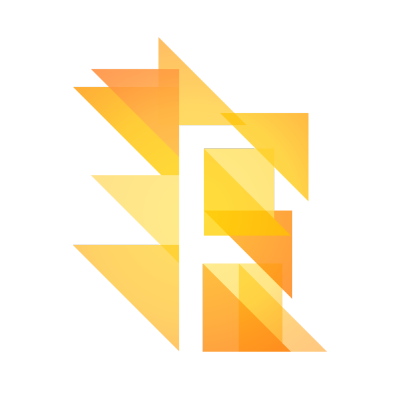
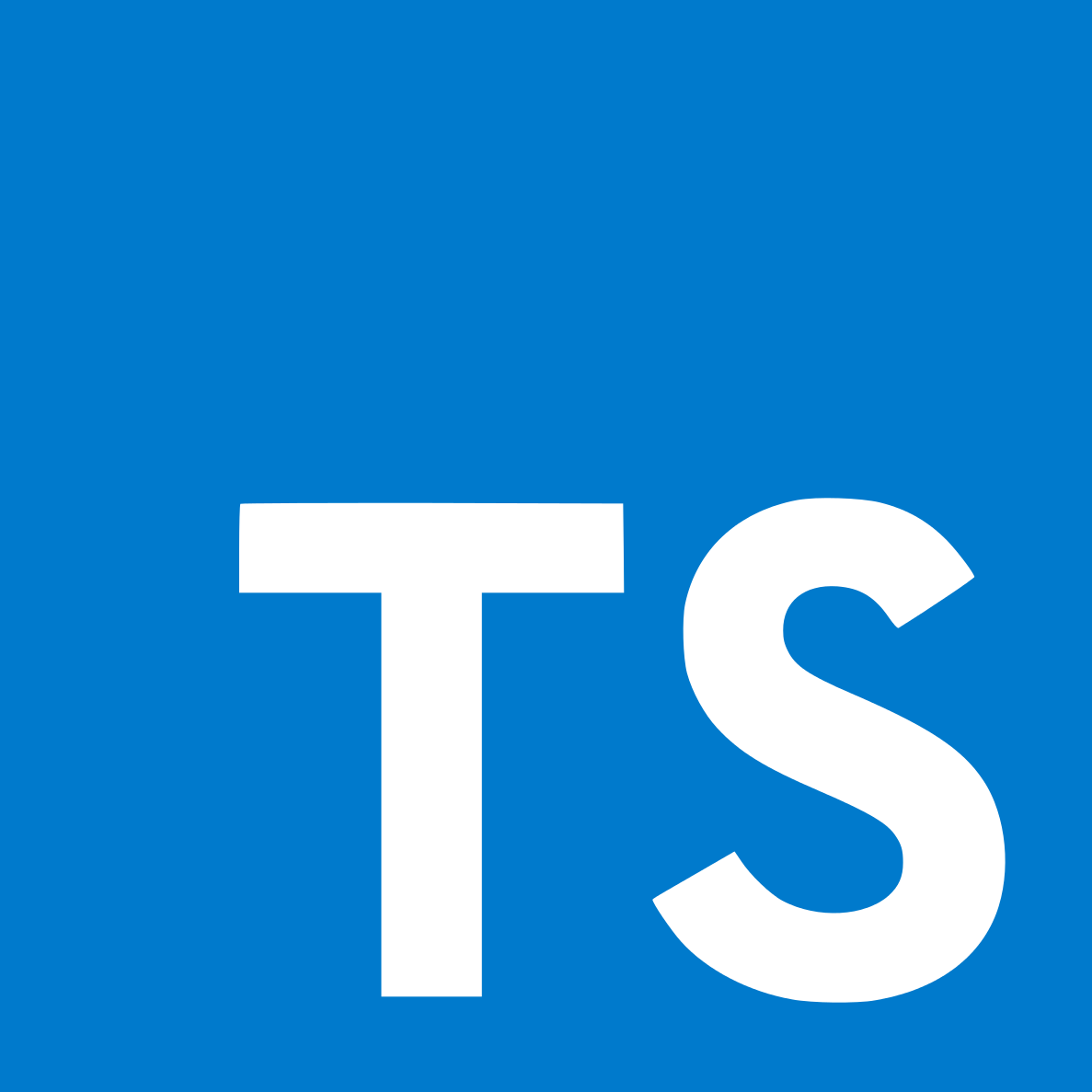
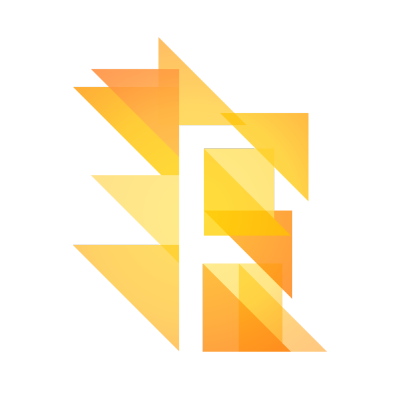
Static Type Checker for JavaScript
...that keeps your sanity
function area (r) {
return Math.PI * r * r
}
const result = area("1O");
// @flow
function area (r) {
return Math.PI * r * r
}
const result = area("1O");
x ^^^^ This type is incompatible with number.
function length (value) {
return value.length
}
const result = length(null);
// @flow
function length (value) {
return value.length
x ^^ property `length`. Property cannot be accessed on possibly null value
}
const result = length(null);
// @flow
function length (value) {
if (value !== null) {
return value.length
}
return 0;
}
const result = length(null);
Changes break applications
// @flow
function showModal(params) {
console.log(params.title);
console.log(params.content);
}
function sayHello (user) {
showModal({
title: `Hello, ${user.name} ${user.surname}!`,
content: 'Welcome to our site!'
})
}
Uncaught TypeError: Cannot read property "name" of undefined!
Quick intro into syntax
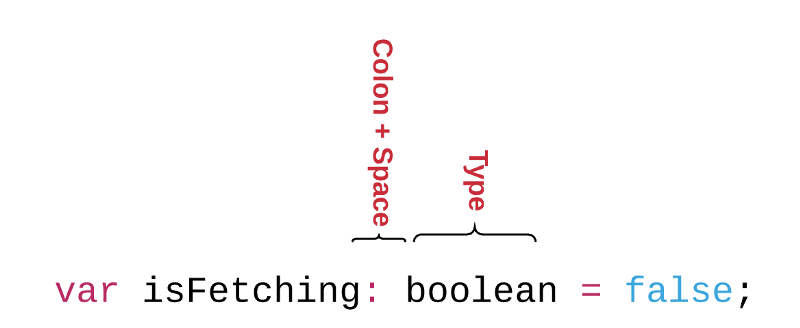
Basic types
-
boolean
-
number
-
string
-
null
-
void
-
Array
-
Object
-
any
-
Function
More features
-
async
-
type aliases
-
generics
-
maybe & exact
-
disjoints
-
imports & exports
-
inference
Setting up Flow
$ npm install --save-dev babel-plugin-transform-flow-strip-types
$ npm install --global flow-bin
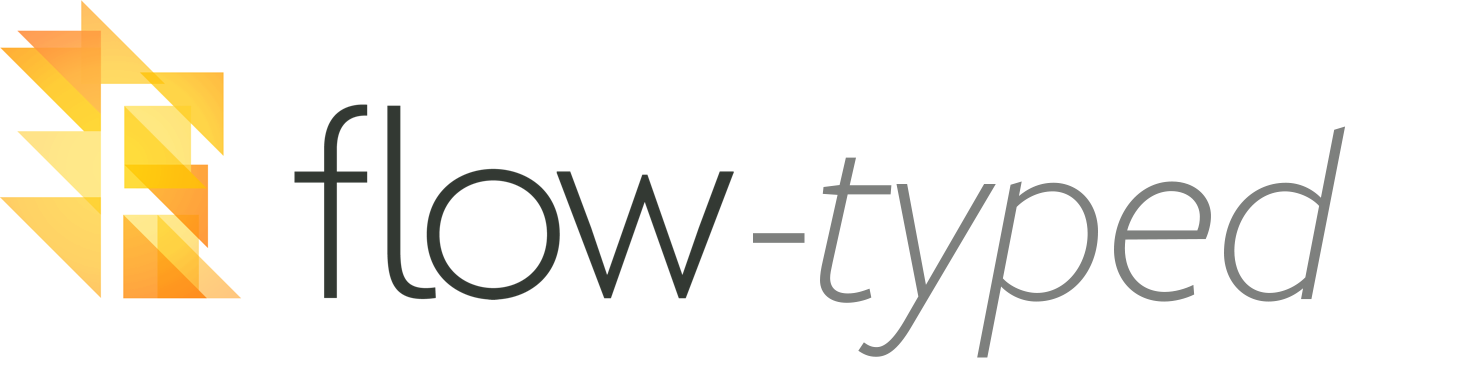
IDE/Editor support
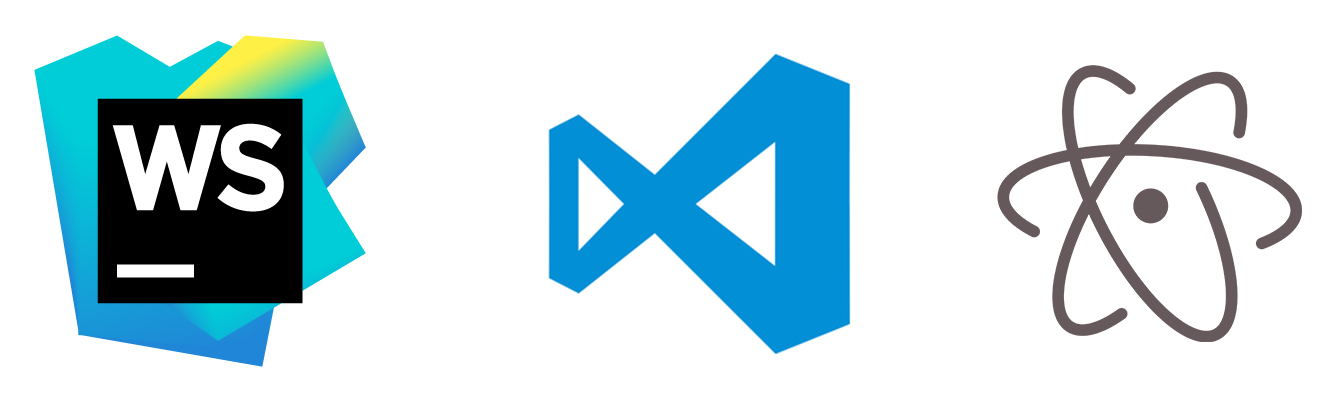
TypeScript vs. Flow
TypeScript
- Compiler + Type Checker
- Has its own parser
- Requires to write more explicit types
- Great support and adoption
- Written in TypeScript by Microsoft
Flow
- Type Checker
- Uses Babel as parser
- Access to Babel infrastructure
- Very good at inferring types
- Written in OCaml by Facebook
Why use typings?
-
Shipping fewer bugs to production
You can detect bugs and errors early. -
Better documentation
Clear containts and preconditions, the way easier to read code. -
Ease of development
Better autocomplete, refactoring with greater confidence -
Other
Reduces excessive error handling and the number of unit tests. Provides a domain modeling tool.
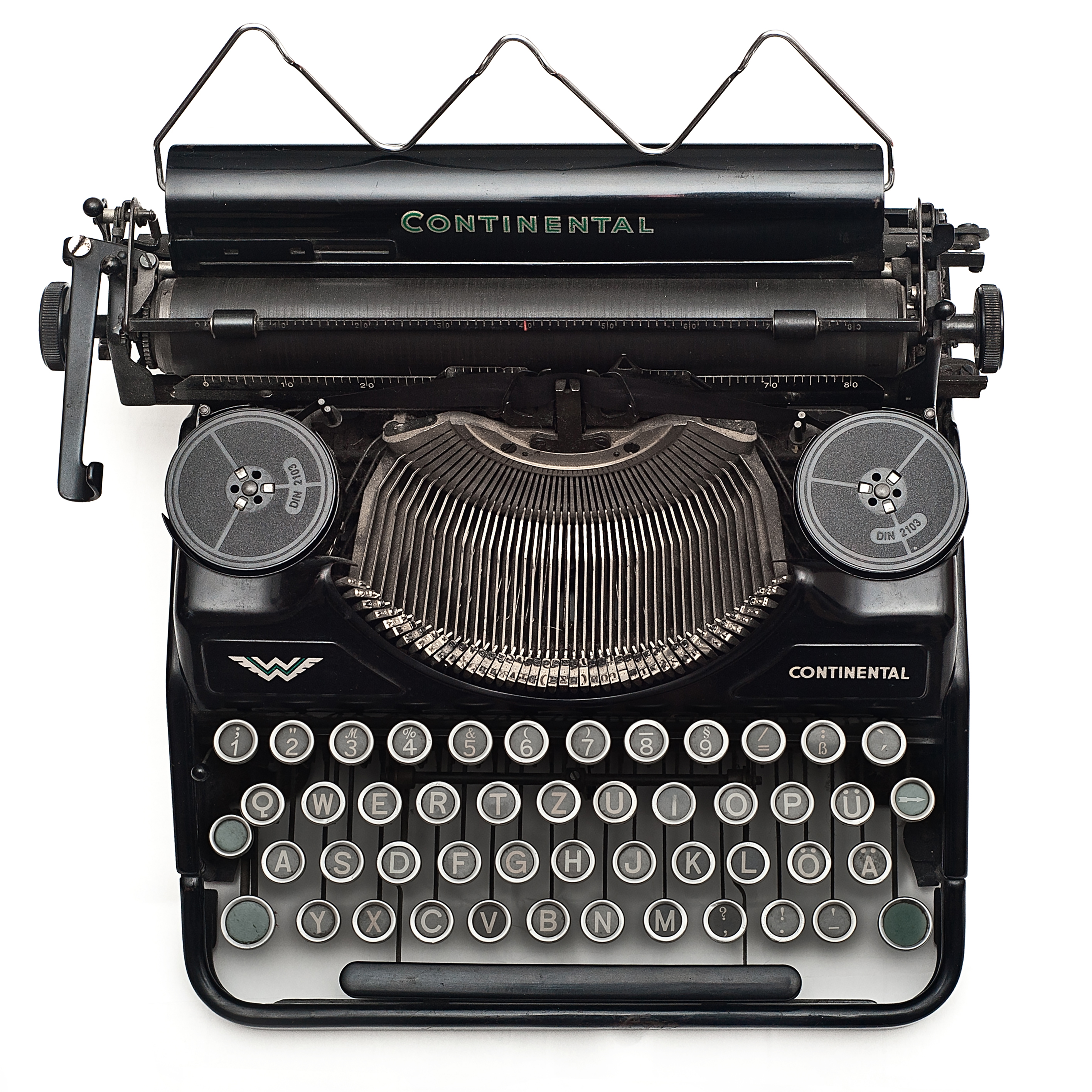
When use typings?
Use
-
The program is critical to business
-
The program is likely to be refactored as your needs evolve
-
The program is complex and has many moving parts
-
The program is maintained by a large team of developers
Don't use
-
The code is short-lived and non-critical
-
You're prototyping and trying to move as quickly as possible
-
The program is small and/or simple
-
You're the only developer
Which should I use?
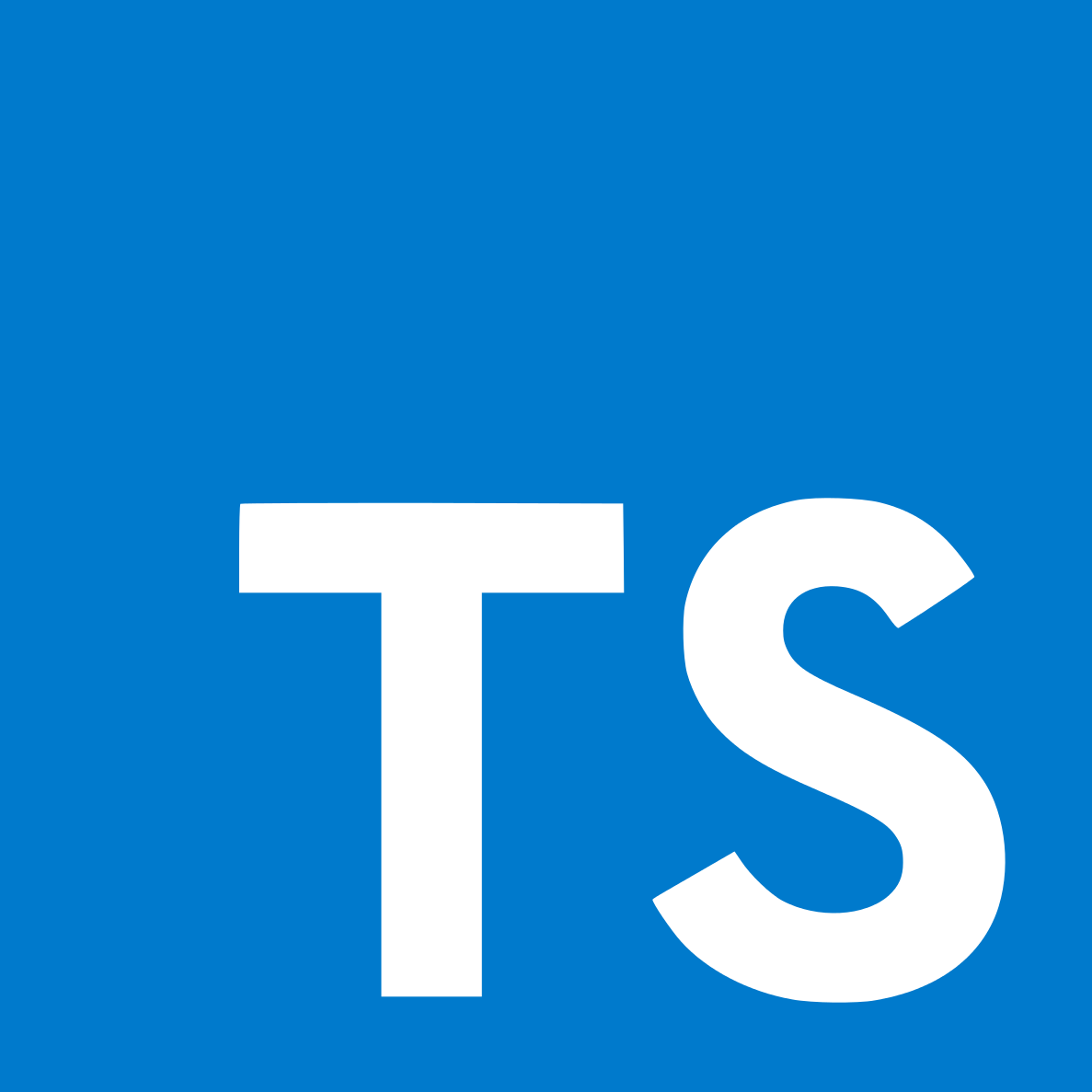
-
You value strong tooling integration
-
You're starting a new project from scratch
-
You're using Angular 2
-
You want to take advantage of more mature typedefs
Which should I use?
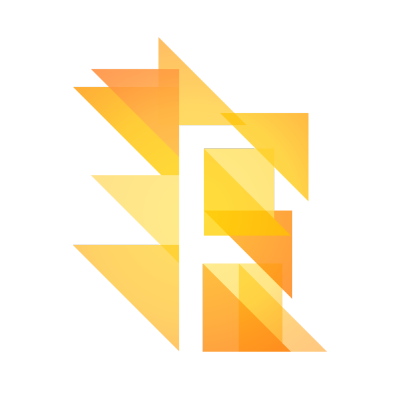
-
You have an existing project
-
You're using React
-
You want to use Babel now or later